SNAP API Documentation
SNAP API DocumentationHow to use API
There are tree ways of using SNAP Universal API.
1. Using universal class cl_nxsAutoPostToSN that allows to post to all (or some) configured networks using settings array.
2. Calling each network’s API connector.
3. Calling each network’s API directlyGoogle+, Instagram, Pinterest, LinkedIn, Fliboard, XING Only) .
Message
This describes the message that could be passed to universal class or API connectors.
Message:
[php]$message = array(
‘pTitle’=>”,
‘pText’=>”,
‘announce’=>”,
‘url’=>”,
‘surl’=>”,
‘urlDescr’=>”,
‘urlTitle’=>”,
‘imageURL’ => array(),
‘videoCode’=>”,
‘videoURL’=>”,
‘siteName’=>”,
‘tags’=>”,
‘cats’=>”,
‘authorName’=>”,
‘orID’=>”
);
[/php]
pTitle – Message title.
pText – Message body.
announce – Message excerpt.
url – URL of the linked or attached page.
surl – Shortened URL of the linked or attached page.
urlTitle – Title of the URL
urlDescr – Description of the URL
imageURL = URL or array [thumb, medium, large, original] – Array of images.
videoCode – code of the youtube video. For example for https://youtu.be/Dy6MpsDPKts code will be Dy6MpsDPKts
videoURL – URL of the video
siteName – Name of the site
tags – Tags
cats – Categories.
authorName – name of the original article author.
orID – ID of the original article
1. Using universal class cl_nxsAutoPostToSN that allows to post to all (or some) configured networks using settings array.
Networks array can be created manually, using “Management Panel” or exported from SNAP WordPress Plugin.
1. Post simple text message to all your configured accounts:
[php]<?php
require_once “../nxs-snap-class.php”;
// First, let’s get settings.
$fileData = file_get_contents(dirname(__FILE__).’/nx-snap-settings.txt’);
$snapOptions = maybe_unserialize($fileData);
if (class_exists(“cl_nxsAutoPostToSN”)) {
// Initialize the class
$nxsAutoPostToSN = new cl_nxsAutoPostToSN($nxs_snapAPINts, $snapOptions);
// Message array contains the post
$message = array(
‘title’=>’Social Networks Auto Poster (SNAP) API’,
‘text’=>’Social Networks Auto Poster (SNAP) API is
a universal API for the most popular social networks’,
);
// Set message
$nxsAutoPostToSN->setMessage($message);
// Make the post
$ret = $nxsAutoPostToSN->autoPost();
}
?>[/php]
2. Post a text message with attached link and specific image ONLY to the first and third Twitter accounts and to the first Facebook account:
[php]<?phprequire_once “../nxs-snap-class.php”;
// First, let’s get settings.
$fileData = file_get_contents(dirname(__FILE__).’/nx-snap-settings.txt’);
$snapOptions = maybe_unserialize($fileData);
$postOnlyTo = array(‘tw’=>array(0, 2), ‘fb’=>array(0));
$snapOptions= nxs_filterOutSettings($postOnlyTo, $snapOptions);
if (class_exists(“cl_nxsAutoPostToSN”)) {
// Initialize the class
$nxsAutoPostToSN = new cl_nxsAutoPostToSN($nxs_snapAPINts, $snapOptions);
// Message array contains the post
$message = array(
‘url’=>’https://www.nextscripts.com/snap-api/’,
‘urlDescr’=>’Social Networks Auto Poster (SNAP) API’,
‘urlTitle’=>’Social Networks Auto Poster API’,
‘imageURL’ => array(
‘large’=>’https://www.nextscripts.com/images/SNAP-Logo_Big_SQ.png’
),
‘title’=>’Social Networks Auto Poster (SNAP) API’,
‘text’=>’Social Networks Auto Poster (SNAP) API is
a universal API for the most popular social networks’,
);
// Set message
$nxsAutoPostToSN->setMessage($message);
// Make the post
$ret = $nxsAutoPostToSN->autoPost();
}
?>[/php]
2. Calling each network API connector directly.
You can also post directly to individual networks using API Connectors. You can do it with or without(Google+, Pinterest, LinkedIn Only) settings array.
Google+
Settings array:
gpUName – Google username
gpPass – Google password
gpPageID – (Optional) Google+ Page ID
gpCommID – (Optional) Google+ Community ID
gpCCat – (Optional) ID of the Google+ Community Category
postType – A or I or T – Type of the Google+ post: “A” – Attached URL, “I” – Image post, “T” – text only post.
Examples:
Using API Connector
[php]<?phprequire_once “nxs-functions.php”; require_once “inc-cl/gp.api.php”;
$message = array(
‘url’=>’https://www.nextscripts.com/snap-api/’,
‘text’=>’Social Networks Auto Poster (SNAP) API is
a universal API for the most popular social networks’,
);
$NToptions = array();
$NToptions[‘gpUName’] = ‘YourUsername@gmail.com’;
$NToptions[‘gpPass’] = ‘YourPassword’;
$NToptions[‘postType ‘] = ‘A’;
$NToptions[‘gpPageID’] = ‘114111009058659350987’;
$ntToPost = new nxs_class_SNAP_GP();
$result = $ntToPost->doPostToNT($NToptions, $message);
if (!empty($result) && is_array($result) && !empty($result[‘postURL’]))
echo ‘<a target=”_blank” href=”‘.$result[‘postURL’].'”>New Post</a>’;
else
echo “<pre>”.print_r($result, true).”</pre>”;
?>[/php]
Direct API Call
Here is the example of how to post a simple message to your Google Plus Business Page Stream: (more examples of using it with Google+ only are here Google+ Automated Posting)
[php]<?phprequire_once “nxs-api/nxs-api.php”;
$email = ‘YourUsername@gmail.com’;
$pass = ‘YourPassword’;
$pageID = ‘114111009058659350987’;
$msg = ‘Post this to Google Plus!’;
$nt = new nxsAPI_GP();
$loginError = $nt->connect($email, $pass);
if (!$loginError)
{
$result = $nt -> postGP($msg, ”, $pageID);
}
else echo $loginError;
if (!empty($result) && is_array($result) && !empty($result[‘post_url’]))
echo ‘<a target=”_blank” href=”‘.$result[‘post_url’].'”>New Post</a>’;
else
echo “<pre>”.print_r($result, true).”</pre>”;
?>[/php]
Settings array:
fbURL – URL of the facebook page. Could be profile, business page or group.
appKey – Facebook APP ID
appSec – Facebook APP Secret
accessToken – Facebook Authorization Token.
pageAccessToken – Facebook Page Authorization Token. (required for posting to business page only)
postType – A or I or T – Type of the Facebook post: “A” – Attached URL, “I” – Image post, “T” – text only post.
attachType – A or S – (“Attached URL” post type only) Type of the attached URL: “A” – Attached, “S” – Shared.
imgUpl – T or A – (“Image” post type only) Where to upload image: “T” – Timeline, “A” – Album.
Here is how to post to Facebook:
Using API Connector
[php]<?phprequire_once “nxs-functions.php”; require_once “inc-cl/fb.api.php”;
$message = array(
‘url’=>’https://www.nextscripts.com/snap-api/’,
‘urlDescr’=>’Social Networks Auto Poster (SNAP) API’,
‘urlTitle’=>’Social Networks Auto Poster API’,
‘imageURL’ => array(
‘large’=>’https://www.nextscripts.com/images/SNAP-Logo_Big_SQ.png’
),
‘pTitle’=>’Social Networks Auto Poster (SNAP) API’,
‘pText’=>’Social Networks Auto Poster (SNAP) API is
a universal API for the most popular social networks’,
);
$NToptions = array();
$NToptions[‘fbURL’] = ‘https://www.facebook.com/MYFBPAGE’;
$NToptions[‘appKey’] = ‘APPID’;
$NToptions[‘appSec’] = ‘SEC’;
$NToptions[‘accessToken’] = ‘user_token’;
$NToptions[‘pageAccessToken’] = ‘page_token’; //## If you are posting to a page
$NToptions[‘postType’] = ‘A’;
$ntToPost = new nxs_class_SNAP_FB();
$result = $ntToPost->doPostToNT($NToptions, $message);
if (!empty($result) && is_array($result) && !empty($result[‘postURL’]))
echo ‘<a target=”_blank” href=”‘.$result[‘postURL’].'”>New Post</a>’;
else
echo “<pre>”.print_r($result, true).”</pre>”;
?>[/php]
Settings array:
appKey
appSec
accessToken
accessTokenSec
Here is how to post to Twitter:
[php]<?phprequire_once “nxs-functions.php”; require_once “inc-cl/tw.api.php”;
$message = array();
$message[‘pText’] = ‘Test Post’;
$message[‘imageURL’] = ‘https://www.nextscripts.com/imgs/nextscripts.png’;
$TWoptions = array();
$TWoptions[‘twURL’] = ‘https://twitter.com/YourTWPage’;
$TWoptions[‘appKey’] = ‘KEY’;
$TWoptions[‘appSec’] = ‘SECRET’;
$TWoptions[‘accessToken’] = ‘TOKEN’;
$TWoptions[‘accessTokenSec’] = ‘TOKENSECRET’;
$TWoptions[‘attchImg’] = ‘1’;
$ntToPost = new nxs_class_SNAP_TW();
$result = $ntToPost->doPostToNT($TWoptions, $message);
if (!empty($result) && is_array($result) && !empty($result[‘postURL’]))
echo ‘<a target=”_blank” href=”‘.$result[‘postURL’].'”>New Post</a>’;
else
echo “<pre>”.print_r($result, true).”</pre>”;
?>[/php]
Blogger
Settings array:
bgUName
bgPass
bgBlogID
bgInclTags
DIIGO
Settings array:
diUName
diPass
diAPIKey
Delicious
Settings array:
dlUName
dlPass
FriendFeed
Settings array:
ffUName
ffPass
grpID
imgToUse
InstaPaper
Settings array:
ipUName
ipPass
Settings array:
ulName
uPass
uPage
grpID
liAPIKey
liAPISec
liOAuthVerifier
liOAuthToken
liOAuthTokenSecret
liAccessToken
liAccessTokenSecret
liAttch
Management panel
(SNAP API PRO only). Upload the contents of the zip file on the server and open snap-setup.php file from the browser. You will see the management panel.
SNAP Universal API
Automatically post from your PHP Projects to all major social networks via one universal API interface.
Get SNAP API
Latest News/Blogposts
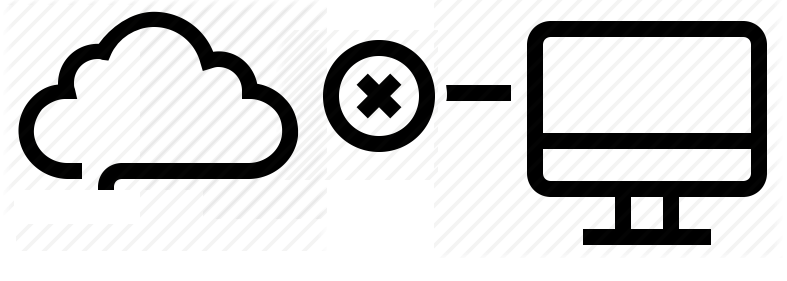
Corrupted cache issue with SNAP Pro.
What happened? SNAP Pro is checking for API update every 6 hours. Today (May 22, 2019) around 6:30PM EST Google Cloud messed our update server for about 7 minutes. A bunch of "lucky" sites that were checking for update during those 7 minutes got their plugin cache...
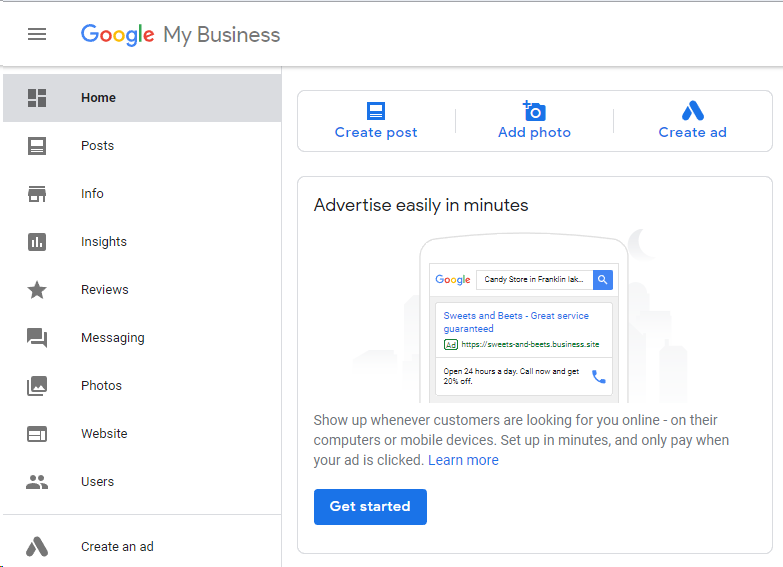
Support for “Google My Business” is coming soon…
Support for "Google My Business" is coming this fall. Google recently made some kind of blog-a-like functionality available for local business listings. As a local business owner you can add posts, events, offers as well as products to your business listing. Although...
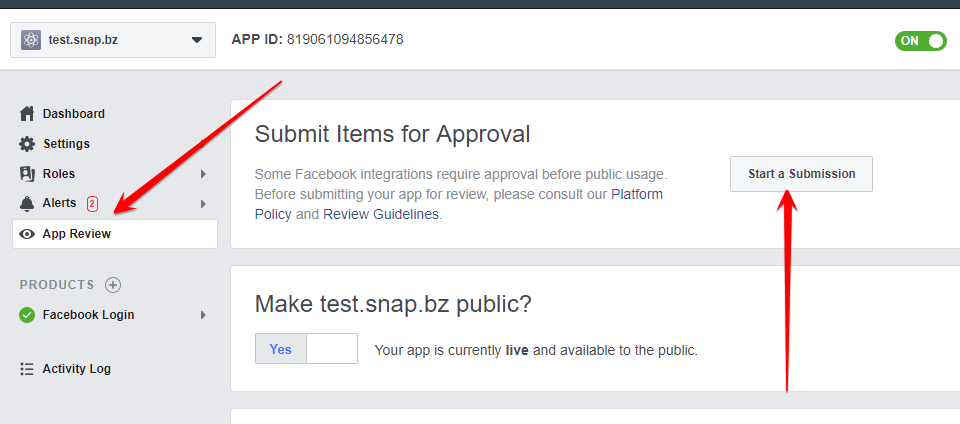
Facebook app review
We are getting a lot of questions about upcoming deadline for Facebook app review. People are asking what should we do. The honest answer would be: "No one has any idea". We submitted several apps for Facebook review using different ways of describing and explaining...
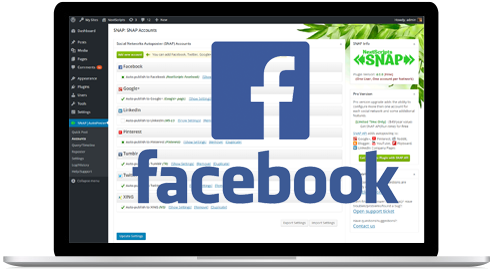
What happened with Facebook
What happened with Facebook? Facebook made changes to it's API access policy on May 1st, 2018. As the result we introduced our own Premium API for Facebook. We feel that we need to explain how exactly those changes affected SNAP. Since the beginning Facebook native...
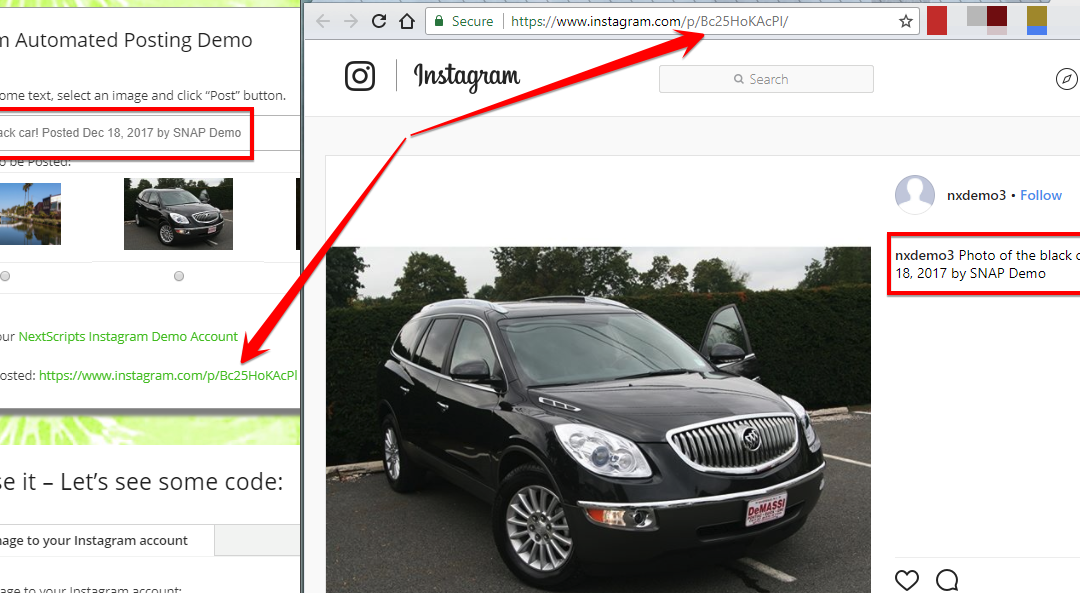
Instagram issues
Instagram made some very big changes to authentication process. About 70% of our users are affected by them. Before the changes the process was quite simple: Sometimes Instagram decided that login from SNAP is "unusual" and asked for confirmation. You just had to open...